Several crucial tasks for the ultimate quality of code are often tedious or even neglected, such as writing unit tests.
Unit testing is generally done by developers under the supervision of Quality Assurance professionals (QAs). However, in the software development cycle, devs sometimes end up doing only basic testing to speed up delivery. With StackSpot, devs can generate more thorough unit tests with a considerable reduction in cognitive load.
This article outlines the main features and advantages of StackSpot AI for generating unit tests and brings a practical example of how to use it in a Java project.
What are unit tests?
Unit tests verify that a program’s code is working correctly by isolating logical units and testing their behavior. They are essential for guaranteeing the quality and reliability of software, as well as facilitating code maintenance and refactoring.
However, writing unit tests can be a tedious and time-consuming task. Most of the time, it is neglected or postponed. That’s why it’s necessary to find tools to automate and simplify this process, generating unit tests from source code or specifications.
One such tool is StackSpot AI.
What is StackSpot AI?
StackSpot AI is a generative artificial intelligence solution that helps optimize the contextualized use of large language models (LLM) to generate high-quality, assertive code. It’s a code assistant that uses your context, knowledge sources, and technological decisions about patterns to produce high-quality, assertive code.
StackSpot AI sets itself apart from other AI solutions by being highly customizable and adaptable to the needs of each company. It also allows you to create quick commands to automate repetitive tasks.
In our case, StackSpot AI allows you to generate unit tests for different programming languages, such as Java, Python, C#, Ruby, and JavaScript. It uses machine learning techniques and static analysis to infer the code’s properties and requirements and generate test cases that cover all possible scenarios.
To use StackSpot AI, visit the website, log in to the AI tool, and download the extension for IDE, Visual Studio Code or IntelliJ.
Below is an example of the plugin installed in Visual Studio Code:
After installation, log in with the exact login details in the AI tool.
Generating unit tests in StackSpot AI
Now, we’ll demonstrate in a practical manner how StackSpot AI can help you generate unit tests.
Here are two examples: the first complementing existing tests by improving coverage, and the other creating a test class from scratch.
Unit tests in StackSpot AI – Example #1
I have a project that only has tests for the Message.java class:
Check out what happens when I run the tests using Pitest to validate the Message.java class test:
public class Message {
public String message(int a) {
String result;
if (a >= 0 && a <= 10) {
result = "YES";
} else {
result = "NO";
}
return result;
}
}
Class: Message.java
public class MessageTest {
@Test
public void messageOk1() {
Message message = new Message();
String result = message.message(5);
assertEquals("YES", result);
}
@Test
public void messageOk2() {
Message message = new Message();
String result = message.message(-5);
assertEquals("NO", result);
}
}
Class: Messagetest.java
After tests using Pitest, the report indicates that the Message.java class has 100% coverage, but the quality of the tests is at 60%. This indicates that we can add more scenarios to cover all possibilities.
Let’s use StackSpot AI to generate the tests and ensure 100% mutation coverage.
Inside the Message.java class, select the code, right-click to open the menu, select “StackSpot AI > Add tests for this code”. Check out the image below:
StackSpot AI processes the information and generates a class with all the tests and a brief code explanation. You can add this entire class to your project or just insert the code into the existing class.
In this example, existing tests have been replaced, and those generated by StackSpot AI were chosen. The MessageTest.java class looks like this:
public class MessageTest {
@Test
public void testMessageWithNumberInRange() {
Message message = new Message();
assertEquals("YES", message.message(5), "The message should be YES for numbers in range 0-10");
}
@Test
public void testMessageWithNumberOutOfRangePositive() {
Message message = new Message();
assertEquals("NO", message.message(11), "The message should be NO for numbers greater than 10");
}
@Test
public void testMessageWithNumberOutOfRangeNegative() {
Message message = new Message();
assertEquals("NO", message.message(-1), "The message should be NO for negative numbers");
}
@Test
public void testMessageWithBoundaryNumberZero() {
Message message = new Message();
assertEquals("YES", message.message(0), "The message should be YES for the boundary number 0");
}
@Test
public void testMessageWithBoundaryNumberTen() {
Message message = new Message();
assertEquals("YES", message.message(10), "The message should be YES for the boundary number 10");
}
}
Class: MessageTest.java
After running the tests generated by StackSpot AI and checking the Pitest report, we can see that all test scenarios for the Message.java class have been covered.
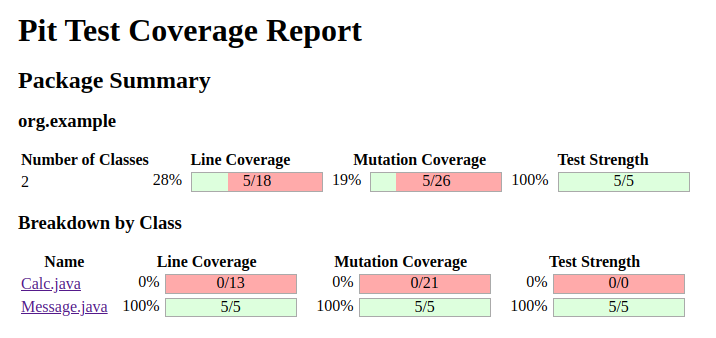
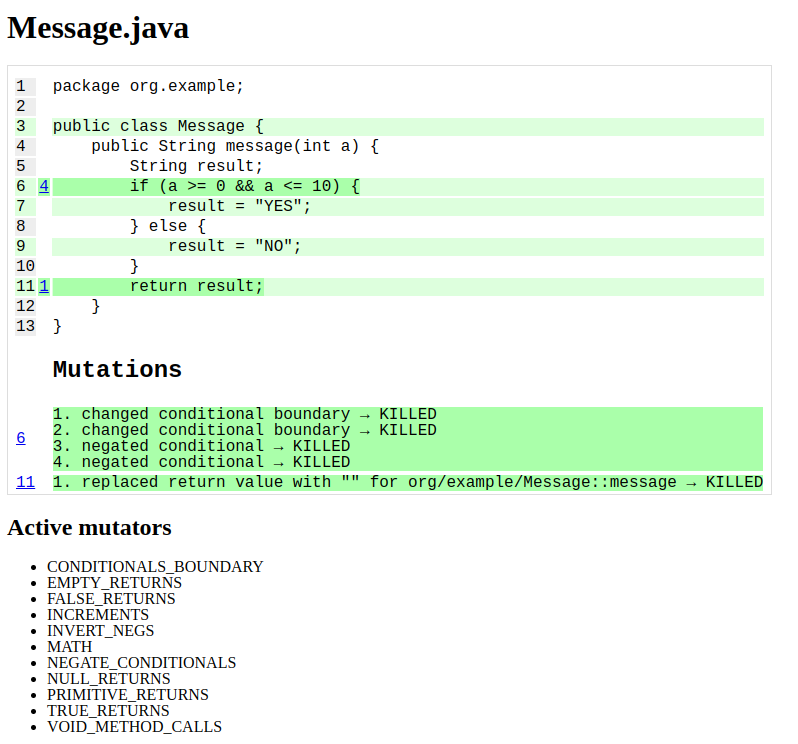
Unit tests in StackSpot AI – Example #2
The same procedure will be applied to the Calc.java class. Let’s create the entire test class in StackSpot AI.
First, select the class code by right-clicking and selecting “StackSpot AI > Add tests for this code” from the menu:
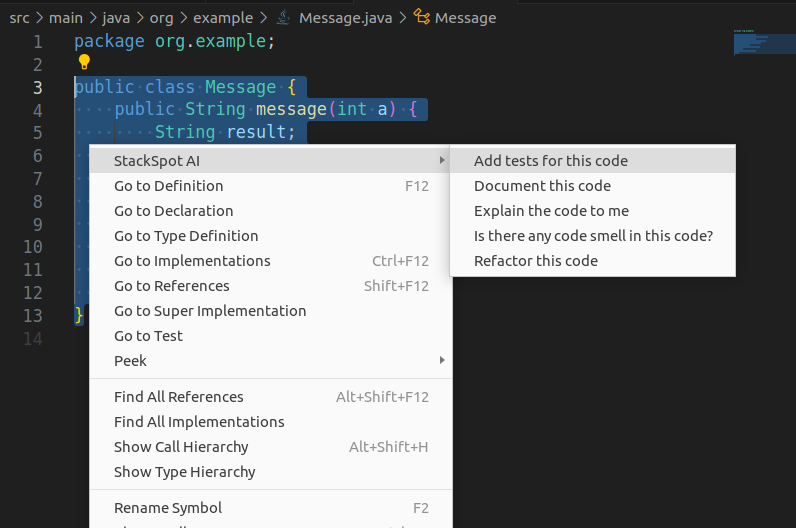
After a few seconds, StackSpot AI generates the test class with all scenarios and code explanations. Check it out:
StackSpot AI generated the CalcTest.java class, which looks like this:
assertNull(Calc.soma(null, 3));
assertNull(Calc.soma(2, null));
assertNull(Calc.soma(null, null));
}
@Test
void testSubtraiWithValidNumbers() {
assertEquals(1, Calc.subtrai(4, 3));
}
@Test
void testSubtraiWithNulls() {
assertNull(Calc.subtrai(null, 3));
assertNull(Calc.subtrai(4, null));
assertNull(Calc.subtrai(null, null));
}
@Test
void testMultiplicaWithValidNumbers() {
assertEquals(12, Calc.multiplica(4, 3));
}
@Test
void testMultiplicaWithNulls() {
assertNull(Calc.multiplica(null, 3));
assertNull(Calc.multiplica(4, null));
assertNull(Calc.multiplica(null, null));
}
@Test
void testDivideWithValidNumbers() {
assertEquals(2, Calc.divide(6, 3));
}
@Test
void testDivideByZero() {
assertNull(Calc.divide(6, 0));
}
@Test
void testDivideWithNulls() {
assertNull(Calc.divide(null, 3));
assertNull(Calc.divide(6, null));
assertNull(Calc.divide(null, null));
}
}
Class: Calctest.java
Tests were rerun, and the report shows that all scenarios met code and mutation coverage.
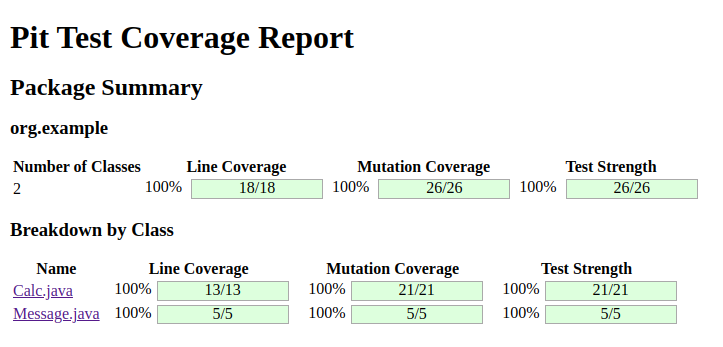
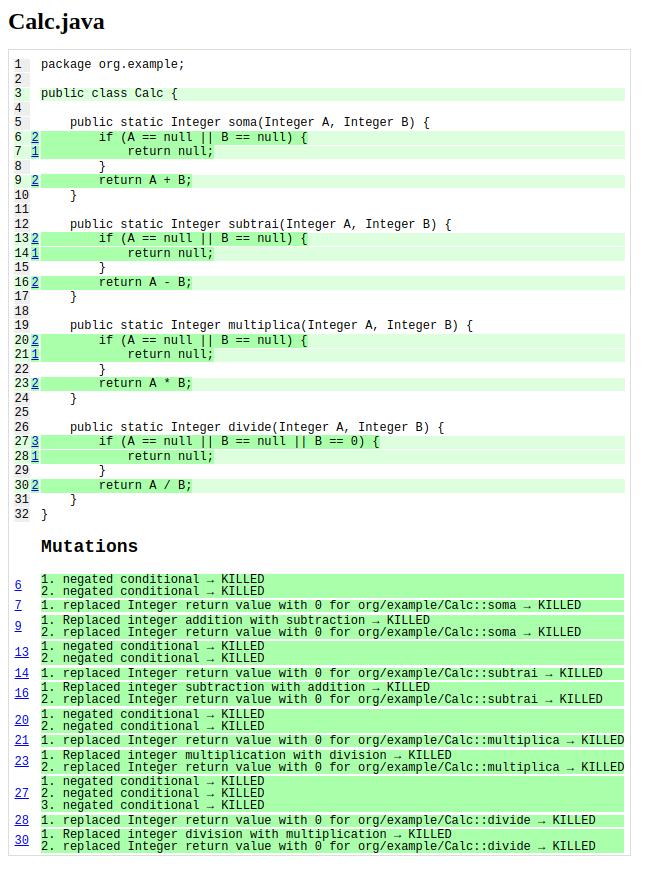
Watch the StackSpot Demos video to see a step-by-step process of how to create unit tests.
Conclusion
StackSpot AI is a powerful and innovative tool that can help developers save time and resources while boosting code reliability and security.
With StackSpot AI, unit tests are generated faster and with higher quality, reducing the team’s cognitive load.
Want to learn more about how to use StackSpot in software development and gain efficiency? Follow posts in the “Tutorials” category of this blog!
Do you have any questions or suggestions? Please leave your comment.
References
- StackSpot AI – Official website
- Getting Started | Docs AI – StackSpot AI Documentation